A recursive functions is a function that makes a call to itself. Recursion is usually used to process tree-like or nested structures with much simpler code than using iterative functions.
This post shows an example in which we need to count the number of files of a folder and each subfolders.
When processed this structure of folders…
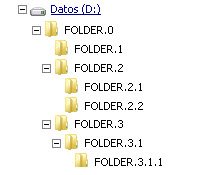
…this is the ouput:
PS D:\Scripting\COUNT-FILES> .\COUNT-FILES.ps1 "D:\FOLDER.0"
NO-SUB SUB Folder
----------------------------------
2 2 D:\FOLDER.0\FOLDER.1
5 5 D:\FOLDER.0\FOLDER.2\FOLDER.2.1
2 2 D:\FOLDER.0\FOLDER.2\FOLDER.2.2
0 7 D:\FOLDER.0\FOLDER.2
3 3 D:\FOLDER.0\FOLDER.3\FOLDER.3.1\FOLDER.3.1.1
0 3 D:\FOLDER.0\FOLDER.3\FOLDER.3.1
0 3 D:\FOLDER.0\FOLDER.3
1 13 D:\FOLDER.0
The first column shows the number of files of each folder.
The second columnd shows the files of each folder including each subfolders.
This is the code you can download here:
param(
[Parameter(Mandatory=$true)][string]$PATH
)
function AnalyzeFolder ($FOLDER)
{
$NUMLOCAL=0
$NUMSUB=0
$FULL = Get-ChildItem "$FOLDER" -Include *.*
Foreach ($ITEM in Get-ChildItem $FOLDER)
{
$FULLNAME=$ITEM.FullName
if (!(Test-Path $FULLNAME -PathType Container))
{
$NUMLOCAL=$NUMLOCAL+1
}
else
{
If ($FULLNAME -ne $FOLDER)
{
$NUMTEMP=AnalyzeFolder "$FULLNAME"
$NUMSUB=$NUMSUB+$NUMTEMP
}
}
}
$NUMTOTAL=$NUMLOCAL+$NUMSUB
$MSG="$NUMLOCAL $NUMTOTAL $FOLDER"
Write-Host $MSG
return $NUMTOTAL
}
Write-Host
Write-Host "NO-SUB SUB Folder"
Write-Host "----------------------------------"
$TOTAL=AnalyzeFolder "$PATH"
Write-Host
- The recursive function (and the only one) is "AnalyzeFolder"
- The recursion is combined with an iteration used inside the function
- This functions processes each item inside the loop: if it's a file it increases the counter and iterates to the next item. If it's a folder, the function calls to itself (the recursive call is bolded in red)
- Note the use of local variables and their combination to summarize the number of files including and without including subfolders