In this post we'll walk through a practical solution—a Bash script that uses SNMP to monitor the state of VPN tunnels in a Check Point VSX environment, specifically designed to integrate with Icinga.
Our Bash script leverages SNMP to check the status of VPN tunnels. Although this example focuses on a VSX environment, the script can be adapted for a standard Check Point deployment with minimal changes.
Below is the complete script:
#!/bin/bash
HOST=$1
VS=$2
PEER_GW=$3
SNMP_USER=$4
SNMP_PASS=$5
SNMP=$(snmpwalk -v 3 -l authNoPriv -u $SNMP_USER -A $SNMP_PASS -n ctxname_vsid$VS $HOST .iso.org.dod.internet.private.enterprises.checkpoint.tables.tunnelTable.tunnelEntry.tunnelState.$PEER_GW.0 2> /dev/null)
if [ -z "$SNMP" ]; then
echo "UNKNOWN: No SNMP response from $HOST"
exit 3
fi
RESULT=$(echo $SNMP | awk '{ print $9 }' 2> /dev/null)
case $RESULT in
3)
echo "OK - VPN tunnel is active (state $RESULT)"
exit 0
;;
4)
echo "Critical - VPN tunnel is destroyed (state $RESULT)"
exit 2
;;
129)
echo "Warning - VPN tunnel is idle (state $RESULT)"
exit 1
;;
130)
echo "Critical - VPN tunnel is during Phase1 (state $RESULT)"
exit 2
;;
131)
echo "Critical - VPN tunnel is down (state $RESULT)"
exit 2
;;
132)
echo "Critical - VPN tunnel is initializing (state $RESULT)"
exit 2
;;
*)
echo "Unknown - VPN tunnel state unknown"
exit 3
;;
esac
- Input Parameters: The script takes five input parameters: the host (IP address or hostname of the Check Point device), the VSID (Virtual System ID), and the peer gateway ID, and the SNMP credentials (USER + PASS)
- SNMP Query: Using
snmpwalk
, the script queries the Check Point device for the VPN tunnel state. The SNMPv3 protocol ensures secure querying. - State Evaluation: The script evaluates the returned state and provides corresponding output messages:
3
: VPN tunnel is active.4
: VPN tunnel is destroyed.129
: VPN tunnel is idle.130
: VPN tunnel is in Phase 1.131
: VPN tunnel is down.132
: VPN tunnel is initializing.
- Exit Codes: The script uses standard Icinga plugin exit codes (
0
for OK,1
for Warning,2
for Critical, and3
for Unknown).
Prerequisites
Before using this script, ensure SNMP is enabled and properly configured on your Check Point gateway. In this example, SNMP v3 with user/password authentication is configured and used to authenticate and query the SNMP.
Test it
After granting proper execution permissions, an example test:
$ ./check_fw_tunnelstate.sh myvsx 2 195.95.95.95 snmp_user snmp_password
OK - VPN tunnel is active (state 3)
Finally, some additional steps are needed to configure it in Icinga, like the command definition, the service definition and reloading the Icinga services so the tunnel is finally monitored:
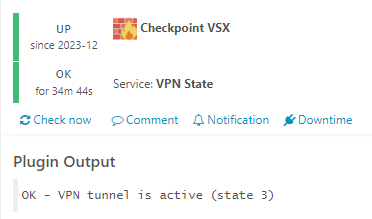